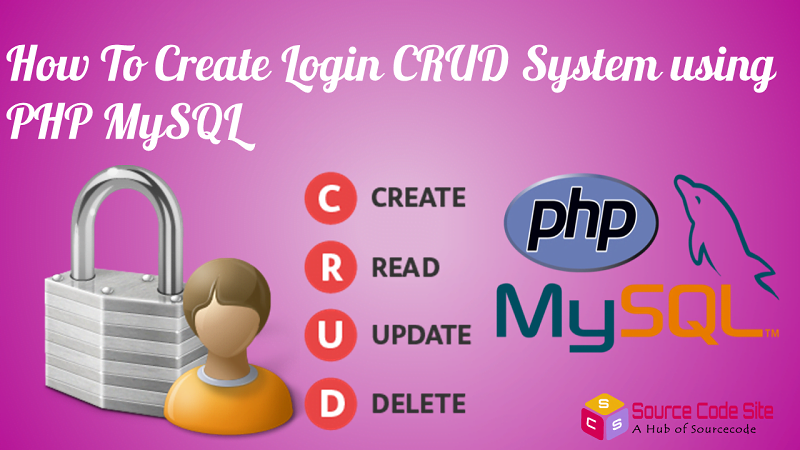
How to Create Login CRUD System using PHP MySQL
Login CRUD System using PHP MySQL Creating CRUD system is a very common task in web development CRUD stand For Create, Read, Update and Delete. if you are a senior web developer you must have created plenty of CRUD system already. They maybe exist in a content management system, and inventory management system OR accounting application. if you just started web development, you are certainly going to experience lots CRUD grid’s creation work in your later career. The main purpose of a CRUD system is that enables users create, read, update, and delete data. Normallay data stored in MySQL Database. PHP is the server side scripting language that manipulates MySQL Database tables to give Front end users power to perform CRUD action. in this post we are creating login system also.
CRUD Operations
C – Create OR Insert data to MySQL Database.
R – Read Database Records.
U – Update Selected MySQL Records
D – Delete Selected Record From MySQL Database.
Create Login CRUD System using PHP MySQL
1-Creating Database
- Open Phpmyadmin in your Browser
- Click on Database Tab Display on Top side
- Give the Database name “quaktis”.
- After Creating Database Open it.
- Click on SQL Tab on Top area
- Copy the Below Source Code and paste it.
- Then Click on Go.
-- phpMyAdmin SQL Dump -- version 4.2.7.1 -- http://www.phpmyadmin.net -- -- Host: 127.0.0.1 -- Generation Time: Jul 11, 2016 at 01:01 PM -- Server version: 5.6.20 -- PHP Version: 5.5.15 SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO"; SET time_zone = "+00:00"; /*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */; /*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */; /*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */; /*!40101 SET NAMES utf8 */; -- -- Database: `quaktis` -- -- -------------------------------------------------------- -- -- Table structure for table `info_tbl` -- CREATE TABLE IF NOT EXISTS `info_tbl` ( `infoID` int(100) NOT NULL, `firstName` varchar(199) DEFAULT NULL, `lastName` varchar(199) DEFAULT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=12 ; -- -- Dumping data for table `info_tbl` -- INSERT INTO `info_tbl` (`infoID`, `firstName`, `lastName`) VALUES (11, 'kumar', 'Deepak'); -- -------------------------------------------------------- -- -- Table structure for table `user_tbl` -- CREATE TABLE IF NOT EXISTS `user_tbl` ( `userID` int(5) unsigned NOT NULL, `username` varchar(30) NOT NULL, `password` varchar(30) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=4 ; -- -- Dumping data for table `user_tbl` -- INSERT INTO `user_tbl` (`userID`, `username`, `password`) VALUES (3, 'admin', 'admin'); -- -- Indexes for dumped tables -- -- -- Indexes for table `info_tbl` -- ALTER TABLE `info_tbl` ADD PRIMARY KEY (`infoID`); -- -- Indexes for table `user_tbl` -- ALTER TABLE `user_tbl` ADD PRIMARY KEY (`userID`); -- -- AUTO_INCREMENT for dumped tables -- -- -- AUTO_INCREMENT for table `info_tbl` -- ALTER TABLE `info_tbl` MODIFY `infoID` int(100) NOT NULL AUTO_INCREMENT,AUTO_INCREMENT=12; -- -- AUTO_INCREMENT for table `user_tbl` -- ALTER TABLE `user_tbl` MODIFY `userID` int(5) unsigned NOT NULL AUTO_INCREMENT,AUTO_INCREMENT=4; /*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */; /*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */; /*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
OR Import DB File
After Downloading the source code extract it in your root folder.
- Open Phpmyadmin in your Browser
- Click on Database Tab Display on Top side
- Give the Database name “quaktis”.
- After Creating Database Open it.
- Click on Import Tab on Top area
- You can Find Db file in Downloaded source code Select it.
- Then Click on Go.
2- Creating Database Connection
After import Database File then next step is creating database connection using php copy the below code and save it is as “conn.php”.
<?php $server = "localhost"; $username = "root"; $pass = ""; $db = "quaktis"; //create connection $conn = mysqli_connect($server,$username,$pass,$db); //check conncetion if($conn->connect_error){ die ("Connection Failed!". $conn->connect_error); } ?>
3 – Create Login Form Using HTML
in this step we are going to create a form where user’s can login easily by provide their name and password.
<form action="index.php" method="POST"> <html> <center><h3>Login Here :</h3></ceter> <table align="center" bgcolor="tan" width="200"> <tr> <td> Username: </td> <td> <input type="text" name="username" required> </td> </tr> <tr> <td> Password: </td> <td> <input type="password" name="pass" required> </td> </tr> <tr> <td align="center" colspan="2" bgcolor="teal"> <input type="submit" value="login" name="log"> </td> </tr> </table> </html> </form>
4 – Check user’s Availability in Database using PHP
in this step we are going to create a script when user’s login thgis script check the MySQL database this user available or not.
<?php include 'conn.php'; session_start(); if(isset($_SESSION['userID'])){ header('location:home.php'); } if(isset($_POST['log'])){ $user = $_POST['username']; $pass = $_POST['pass']; $sql = "SELECT * FROM user_tbl where username = '$user' and password = '$pass'"; $result = $conn->query($sql); if($result-> num_rows > 0){ while($row= $result->fetch_assoc()){ $_SESSION['userID'] = $row['userID']; $_SESSION['username'] = $row['username']; } ?> <script> alert('Welcome <?php echo $_SESSION['username']?>'); </script> <script>window.location='home.php';</script> <?php }else{ echo "<center><p style=color:red;>Invalid username or password</p></center>"; } $conn->close(); } ?>
5 – Create Home Page After Login
in this step we are goint to create a page after login this page will display.
<?php include 'session.php'; $username = $_SESSION['username']; $userID = $_SESSION['userID']; ?> <!DOCTYPE html> <html> <head> <link rel="stylesheet" type="text/css" href="mycss.css"> <title> This is Sample </title> </head> <body> <div id="body"> <div id="menu"> <ul> <li><a href="home.php">Home</a></li> <li><a href="maintenance.php">Maintenance</a></li> <li><a href="logout.php">Logout</a></li> </ul> </div> <div id="content"> <h1>Welcome <?php echo $username;?></h1> <h3 style="color:blue;"><?php echo "Today is". " ". date("M/d/y") . " - " . date("l") . ""?> <?php echo "" . date("h:i:sa") . ""?></h3> <hr style="border:1px solid red;" > </div> </div> </body> </html>
6 – Creating a Form and insert data into database using PHP
in this step we goint start CRUD system and first step of CRUD system is Creating or inserting data into databse.
<?php include 'conn.php'; include 'session.php'; if(isset($_POST['add'])){ $fname = $_POST['fname']; $lname = $_POST['lname']; $insert = "insert into info_tbl (firstName,lastName) values ('$fname','$lname')"; if($conn->query($insert)== TRUE){ echo "Sucessfully add data"; header('location:maintenance.php'); }else{ echo "Ooppss cannot add data" . $conn->connect_error; header('location:maintenance.php'); } $insert->close(); } ?> <html> <head> <link rel="stylesheet" type="text/css" href="mycss.css"> <title> This is Sample </title> </head> <body> <div id="body"> <div id="menu"> <ul> <li><a href="home.php">Home</a></li> <li><a href="maintenance.php">Maintenance</a></li> <li><a href="logout.php">Logout</a></li> </ul> </div> <div id="content"> <form action="result.php" method="get" ecntype="multipart/data-form"> <table align="center"> <tr> <td>Search: <input type="text" name="query"><input type="submit" value="Search" name="search"></td> </tr> </table> </form> <form action="maintenance.php" method="POST"> <table align="center"> <tr> <td>Fisrt Name: <input type="text" name="fname" value="" placeholder="Type Firstname here" required></td> </tr> <tr> <td>Last Name: <input type="text" name="lname" placeholder="Type Last Name here.." required></td> </tr> <tr> <td><input type="submit" name="add" value="Add"></td> </tr> </table> </form> <br> <table align="center" border="1" cellspacing="0" width="500"> <tr> <th>First Name</th> <th>Last Name</th> <th>Action</th> </tr> <?php $sql = "SELECT * FROM info_tbl"; $result = $conn->query($sql); if($result->num_rows > 0){ while($row = $result->fetch_array()){ ?> <tr> <td align="center"><?php echo $row['firstName'];?></td> <td align="center"><?php echo $row['lastName'];?></td> <td align="center"><a href="edit.php?infoID=<?php echo md5($row['infoID']);?>">Edit </a>/<a href="delete.php?infoID=<?php echo md5($row['infoID']);?>">Delete</a></td> </tr> <?php } }else{ echo "<center><p> No Records</p></center>"; } $conn->close(); ?> </table> </div> </div> </body> </html>
7 – Retrieve Inserted data on Page From Database
this is Second step of CRUD system means Read or Retrieve Mysql Database data .
<table align="center" border="1" cellspacing="0" width="500"> <tr> <th>First Name</th> <th>Last Name</th> <th>Action</th> </tr> <?php $sql = "SELECT * FROM info_tbl"; $result = $conn->query($sql); if($result->num_rows > 0){ while($row = $result->fetch_array()){ ?> <tr> <td align="center"><?php echo $row['firstName'];?></td> <td align="center"><?php echo $row['lastName'];?></td> <td align="center"><a href="edit.php?infoID=<?php echo md5($row['infoID']);?>">Edit </a>/<a href="delete.php?infoID=<?php echo md5($row['infoID']);?>">Delete</a></td> </tr> <?php } }else{ echo "<center><p> No Records</p></center>"; } $conn->close(); ?> </table>
8 – Update inserted Data using PHP
This is the Third step of CRUD system Update or Edit Data.
<?php include 'conn.php'; include 'session.php'; $id = $_GET['infoID']; $view = "SELECT * from info_tbl where md5(infoID) = '$id'"; $result = $conn->query($view); $row = $result->fetch_assoc(); if(isset($_POST['update'])){ $ID = $_GET['infoID']; $fn = $_POST['fname']; $ln = $_POST['lname']; $insert = "UPDATE info_tbl set firstName = '$fn', lastName = '$ln' where md5(infoID) = '$ID'"; if($conn->query($insert)== TRUE){ echo "Sucessfully update data"; header('location:maintenance.php'); }else{ echo "Ooppss cannot add data" . $conn->error; header('location:maintenance.php'); } $conn->close(); } ?> <html> <head> <link rel="stylesheet" type="text/css" href="mycss.css"> <title> This is Sample </title> </head> <body bgcolor="green"> <div id="body"> <div id="menu"> <ul> <li><a href="home.php">Home</a></li> <li><a href="maintenance.php">Maintenance</a></li> <li><a href="logout.php">Logout</a></li> </ul> </div> <div id="content"> <form action="" method="POST"> <table align="center"> <tr> <td>Fisrt Name: <input type="text" name="fname" value="<?php echo $row['firstName'];?>" placeholder="Type Firstname here" required></td> </tr> <tr> <td>Last Name: <input type="text" name="lname" value="<?php echo $row['lastName'];?>" placeholder="Type Last Name here.." required></td> </tr> <tr> <td><input type="submit" name="update" value="Update"></td> </tr> </table> </form> <br> </div> </div> </body> </html>
9 – Delete Data using PHP
This is the final step of CRUD system means Delete or Remove Data using PHP.
<?php include 'conn.php'; $id = $_GET['infoID']; $sql = "Delete from info_tbl where md5(infoID) = '$id'"; if($conn->query($sql) === true){ echo "Sucessfully deleted data"; header('location:maintenance.php'); }else{ echo "Oppps something error "; } $conn->close(); ?>
10 – Creating the Session using PHP
in this step we are going to create session of login.
<?php session_start(); if(!isset($_SESSION['userID']) || (trim($_SESSION['userID']) == '')){ header('location:index.php'); exit(); } $session_id = $_SESSION['userID']; $session_id = $_SESSION['username']; ?>
11 – Creating Logout
in this step we are going to create Logout for login user’s.
<?php session_start(); session_destroy(); unset($_SESSION['userID']); header('location:/sample/index.php'); exit(); ?>
If you facing any type of problem with this source code then you can Download the Complete source code in zip Formate by clicking the below button Download Now otherwise you can send Comment.
I tried to copy the code from screen but didnt understand which code is for which file.
I tried to download source code but also not possible.
you made it difficult to understand
plz check
Great work, but I can not download the source code.
plz check
It should be fine if you use the download button and correctly import the DB. After that startup your XAMPP -> Apache and MySQL and it all should work fine.
Hello Deepak Raj. This is a nice breakdown. Although for a newbie, I am unable to understand which file goes where and unfortunately, I am unable to download the sample zip file.
https://www.sourcecodessite.com/wp-content/uploads/2016/09/Sample.rar